The ultimate C# course with EZTyping
by: Jarne DirkenChoosing a programming language to dedicate your time to can be difficult. This is a difficult decision to make even for seasoned coders. When you're a rookie developer, though, deciding which programming language to learn first might be much more difficult. There are so many to pick from that it might be difficult to understand what each one is for.
While there's a lot to be said for diving in and getting started, this isn't always the case when it comes to picking a programming language to learn. Learning a new language takes time, so it's best to familiarize yourself with its benefits and applications before beginning your studies. Continue reading to discover the benefits of learning C# and then why programmers choose it.
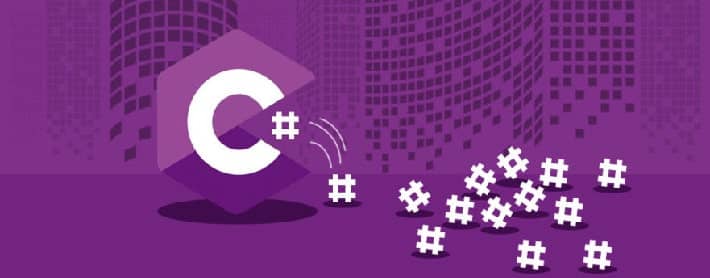
Why Learn C#?
Microsoft's most popular programming language is C#. It was created almost 20 years ago, and with each new release, its popularity has grown much more. The following are some of the reasons why developers prefer C#:
- C# is a high-level programming language. This means it handles a lot of the code that would otherwise be written in languages like Java or C++. It also includes libraries that enable developing code faster and easier.
- C# is a popular programming language. Thousands of businesses use Microsoft technology in their infrastructure, and C# remains a popular language for developing web services even when they don't.
- C# is now cross-platform and open-source. C# was created especially for Windows when it was first released, but it is now also available for Mac OSX and Linux.
- C# is a reasonably simple language to learn. A developer doesn't have to stress about these potential concerns ahead of time because it omits many of the more complex jobs in programming.
- C# is lightning quick. It's easier to write quick code with this language since it's statically typed, and it can handle larger jobs more efficiently. To put it another way, C# allows you to develop high-performance apps quickly.
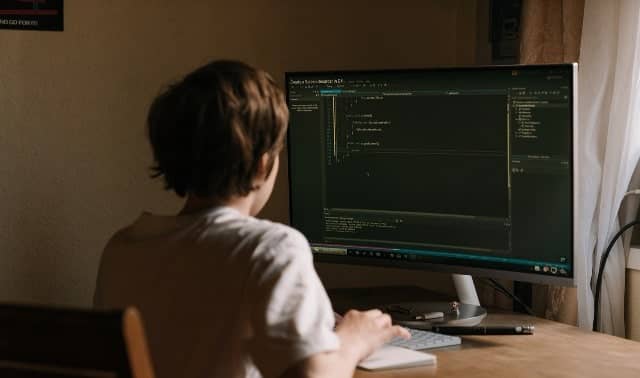
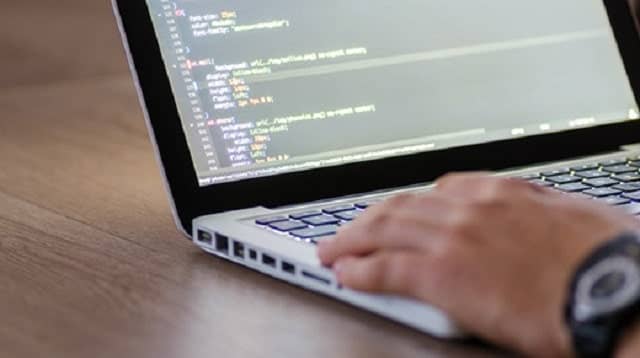
Requirements
- A computer - Windows, Mac, and Linux are all supported.
- A IDE (Integrated development environment). We recommend Visual Studio Code because it's completely free and easy to use!
- C# itself. You can install it inside your IDE.
- .NET Framework 4.8. You can install it on the Microsoft website.
- Your enthusiasm to learn this go-to programming language. It’s a valuable lifetime skill which you can’t unlearn!
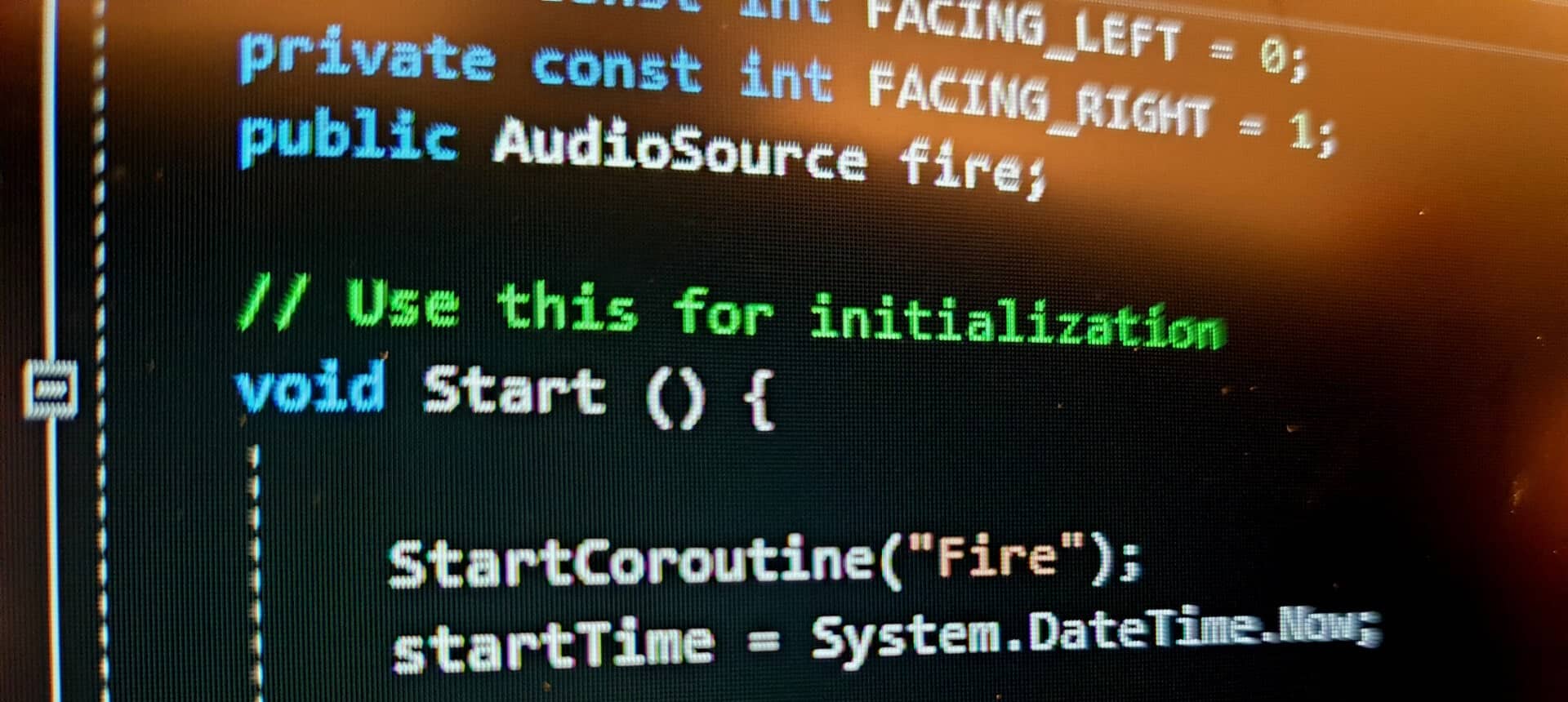
Game Development
C# isn't really used to develop games on it's own. Almost all the time it's used in game engines. Famous game engines that use c# are: Unity, Cryengine and Godot. A top benefit of c# is that c# has compatible frameworks and great tools to improve C#’s game-building capacity.
Basics
- Print statements
Print statements in c# are fairly simple to understand. The biggest difference between python and c# is the environment. In python you can create a .py file and start programming, with c# you can't. You simply type "Console.WriteLine" with parentheses. In those parentheses you type your double quotes, in between you put the text you want to print out.
- Input statements
Asking an input from the user is also quite easy in c#. First you ask your question and than you make a variable and use the Console.ReadLine() method to save that variable.
- If statements
If statements are a little more complex but still doable so pay attention. First we make a variable, we call it specialNumber. Than we ask the user to enter a number ant save this number in a variable "number". Because c# doesn't automatically convert Strings (which is the default input) to numbers, we have to do this ourself with the Convert.ToInt32() method. Now the if statement. We check if our specialNumber is the same ("==") as the number we just entered. If it is the same we print "Goodjob! You guessed the number". If it is not the same we print "Try again..."
- While loops
Loops in c# are more on the complex side but we will do our best to explain everything in as much detail as possible. First we talk about while loops. These loops will keep running as long as the statement between the brackets is met.
So in this example we created a variable named "number" and we gave it a value of 1. Now we say while our number is smaller than 4 keep doing something. That something is printing out our variable number and adding the value 1 to the number. This means the first time it's going to loop it will print out 1 because that is the variable of "number" and right after that it's going to add 1 to our variable. So the next time the value of our "number" is going to be 2. This keeps happening until the statement isn't true anymore. - For loops
Now we are going to talk about for loops in c#. These are a little more difficult to understand than while loops but it works the same. We are going to work with the same example as we did with the while loop.
We create a variable called "Number" and give it a value of 1. You can see that the statement in the for loop has 3 portions.
In the first portion we say for (number) This means we use number. In the second part is the condition so (number < 4). The third part is what is going to happen after the for loop, we are going to add a value of 1 each time our loops ends (number++).